LAB 08B - SMFL FUN
Concepts
For this assignment, you have the opportunity to play with the power that SFML (a special framework called Simple and Fast Multimedia Library) offers. Your solution to this lab assignment will be submitted with Homework 08.
SFML
SFML is a multimedia Application Programming Interface (API) written in C++ with bindings for various programming languages, including Java, Python, and Ruby. SFML provides an easy way to write code that requires graphics and/or sound effects. SFML is the chosen platform for many cool games, including the Atom Zombie Smasher. You can check out everything SFML has to offer by reading the SFML documentation.
Before you Begin
For this specific homework you should download this project template that has initial code and other resource files for you to get started. This file should work with any visual studio installation (on campus or on your home computer). Start the extraction process now, and then read the rest of these instructions (as the extraction process might take some time). Say what? How do I "extract"?
First, save the file linked above to your Z drive
Note: we will always assume you are working on a Mines computer. It is your responsibility to modify these instructions if not.
After you've downloaded the zip file, you need to extract the contents. (We always use Windows Explorer to move and extract contents of a zip file.) You can extract the contents of the zip file anywhere onto your Z drive, but it must be on your Z drive (might as well put it into your CSCI261 folder).
- Click on the Start button and select Computer in the middle of the right side of the menu. This opens Windows Explorer, not to be confused with Internet Explorer.
- Navigate to the zip file downloaded previously.
- Right-click on the zip file and choose Extract All... from the pop-up menu.
- In the dialog that opens, delete what is there and type Z:\
Again, you may specify any folder on your Z drive (other than the adit folder), but it must be on your Z drive. If you want to place in your CSCI261 folder, then type the following for the extraction folder:
Z:\CSCI261\
- Click Extract. Depending on your system's configuration, you may be asked whether you want to copy the extraction process without encryption. If you are asked, mark the option ''Do this for all current items'' and click YES. See the example below.
- Then, using Windows Explorer, rename the project folder to Lab08B.
The next step is the most important in the process ... please be careful!
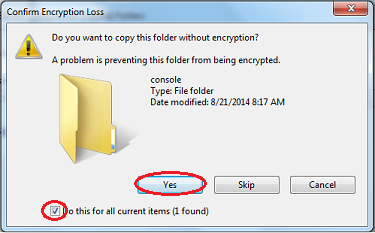
Open and Run the Project
- Launch Visual Studio, if you haven't already.
- When the IDE is loaded, pull down the FILE menu, expand the Open sub-menu, and select Project/Solution...
- Navigate to the Lab08B folder created previously.
- Select sfml-template.sln and click the Open button.
- Now, right click on Solution 'sfml-template' and rename the Solution to Lab08B. Do the same thing for the Project.
Instructions
First, take a look at the main.cpp
file provided in the
SFML template. In class, we discussed each of the commands
shown (e.g., creation
of the window object and the polling for events);
ask questions if there is any confusion.
Second, we also saw the development of a smiley face in class today. The complete code is available here. A few key lines of code covered follow:
CircleShape star;
star.setPosition( 15, 15 );
star.setRadius( 300 );
star.setFillColor( Color::Yellow );
window.draw( star );
// Draw a rectangle object called rect and color it blue
RectangleShape rect;
rect.setSize( Vector2f( 45, 150 ) );
rect.setPosition( 200, 150 );
rect.setFillColor( Color(0, 0, 255) );
window.draw( rect );
// Draw a text object called label
Font myFont;
if( !myFont.loadFromFile( "data\\arial.ttf" ) )
return -1;
Text label;
label.setFont( myFont );
label.setString( "Hello World!" );
label.setPosition( 250, 520 );
label.setColor( Color::Black );
window.draw( label );
Your job is to draw something in SFML. What you draw can be anything you want except a smiley face (e.g., a tree, a bike, a dog, a word using rectangles/circles, etc.). For full credit, you must draw at least five objects. Be creative and have fun!
Possible Extra Credit
You can earn 2 points of extra credit if the item you draw actually moves (yes, creates a video - awesome!). Movement in SFML is actually easier than you might think. As you know, you add all your draw commands in the window loop:
// ADD SEVERAL DRAW COMMANDS HERE
// Apply all the draws to the screen
window.display();
}
Remember your smiley face solution for Homework 01? You've sure come a long way since then! Do you recall how we had a small prize for the best smiley face submitted in each section (Mines frisbee), and a larger prize for the best smiley face submitted in all sections (a 25 point HW)? That was cool. Let's do this again for Lab 08B. That is, we'll have a small prize for the best Lab 08B submitted in each section and a larger prize for the best Lab 08B submitted in all sections. Good luck!