This assignment is due by Friday, September 16, 2016 by 11:59pm.
The town has become quiet and you have trouble finding anyone else that arrived on the ship with you. From what you can piece together,
it seems almost everyone has left the town. Determined to find companions, you venture out of town.
You travel for three days since leaving town and have not seen another soul. The terrain has
been difficult to navigate and you have grown tired. Deciding to stop for the evening, you set up camp and drift asleep.
You awake to find a caravan passing by. The sun is high and you fathom you've been sleeping for some while now. As
the group makes their way along the road, a man breaks rank and walks toward you.
"Hark! We have been looking for you. We passed through a town a few days back heard
said you were already on your way. We are glad to have finally found you. We wish to aid you in your journey.
We would like to offer you one of our transports. Surely you do not want to continue on foot much longer."
You do not immediately respond to the stranger. Walking has given you much time to reflect on your
upcoming challenges, but a part of you wants to get to another village soon and spend a night in an actual bed.
As you continue to think, you ask the man to sit and join you.
Part I - Give Your Hero Life
The man must realize this is a difficult decision for you. (Can I trust the man? Will the transport
prove to be more trouble than it's worth?) He passes you a book, "The Guide to Transports," and you
begin to flip through the pages.
For this assignment, you are to create an OpenGL/GLUT program that contains either your Hero or a vehicle to transport your
Hero drawn in 3D. Be sure to use Lab02 as the basis of your program. The user will be
able to move the 3D character forward and change its heading, as well as control a camera which rotates around the character.
The character itself will be constructed of GLUT primitives and GLU quadrics in a hierarchical fashion, like the previous
assignment. The character must have some level of animation when moving and may also have a different form of animation when stopped.
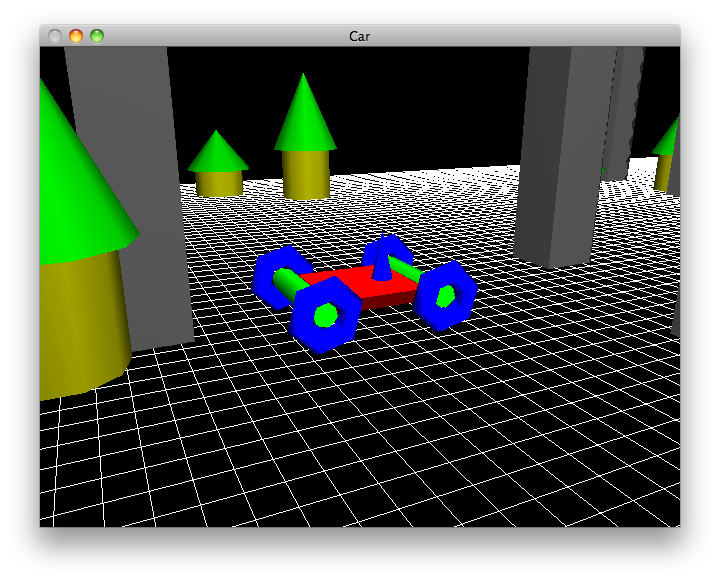 Out for a Sunday drive through the city/park.
Your character should have a clear front and back which are aligned with its current heading. Pressing 'w' will move the character
forward along the heading and pressing 's' will move the character backwards along the heading. Pressing 'a' or 'd'
should turn the character's heading left or right respectively. The user must be able to control an arcball camera with the
mouse that is centered around the character (looking at the character's current X, Y, Z). In other words, the user can
change theta and phi to rotate the camera around the character as the character moves and the character will stay in the center of the
screen.
Additionally, there must be some other objects in the scene so that we can see the character moving. You can now bring your world to life!
At a minimum, this should include a grid of line strips and additionaly can be as sophisticated as a city or forest. You do not need to
worry about checking if your character has collided with one of these objects. For an added challenge though, feel free to add some simple
collision detection to prevent your character from running through every object. Your character should not be
able to move off the grid. Bounds checking should be performed to keep the
character in its world. If you are feeling particularly adventurous, add hills and valleys to your world map. However, if your
world has changes in elevation then your character must also move vertically with the elevation change.
Be creative! Your character can be a 3D humanoid representation of your Hero or a vehicle to transport your Hero.
The vehicle does not need to be a car, but any type of transportation device (wagon, helicopter, plane, scooter, rocketship, etc).
It would be a wise idea to have a function drawCharacter() that does all of the hierarchical drawing for your character. You
will be using this character again in future assignments.
For students in CSCI441: This next section is for the extra credit achievement. For students in CSCI598B: This next section is REQUIRED.
Using viewports, render additional camera views, and overlay them
on top of the normal 3D view. Your viewport may occupy any portion of the space on the screen; you could make the viewport split-screen
style or have it occupy a corner of the screen like a mini-map. In order to receive full credit, you MUST have multiple objects
making up a scene (i.e., boxes of different size as a city, cones stacked on top of cylinders for trees, etc.). In other words, the scene
must be more complicated than just a grid for the ground.
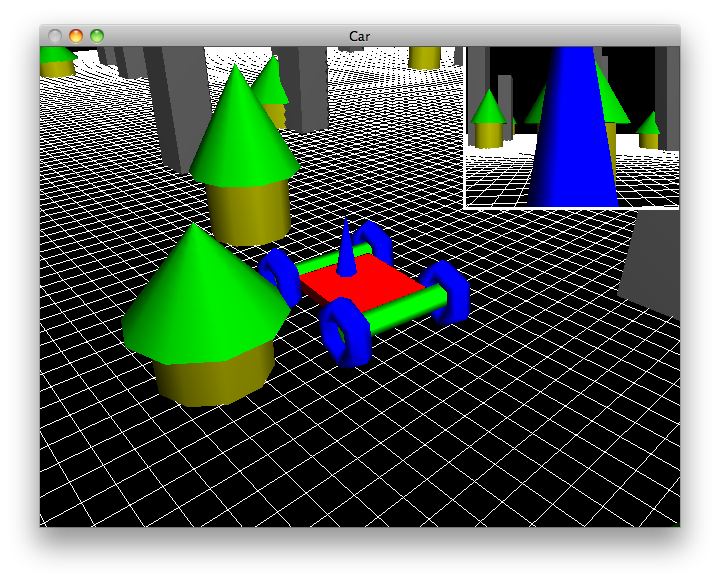 Watch out for that tree!
If you want to implement additional camera models beyond the arcball camera, here is a list of camera models you can
choose from:
- First-Person Cam: The view looking from the point-of-view of the character along its heading.
- Sky Cam: A camera positioned directly overhead the character and looking straight down. The camera must follow
the character and turn with the character so that the character is always pointing forward (towards the top of your window).
- Third-Person Cam: A camera that is located just behind the character and looking along the character's heading. The
camera must follow the character and stay a fixed distance behind the character. (Think Mario Kart)
- Reverse Cam: Similar to the third-person cam, but instead is positioned in front of the character and looking backwards
along the character's heading.
- First-Person Movable Cam: Position as described by the first-person camera, but the user can control the direction of view
to mimic the character looking around the world.
The user should be able to toggle between any available cameras by pressing the 1-9 number keys.
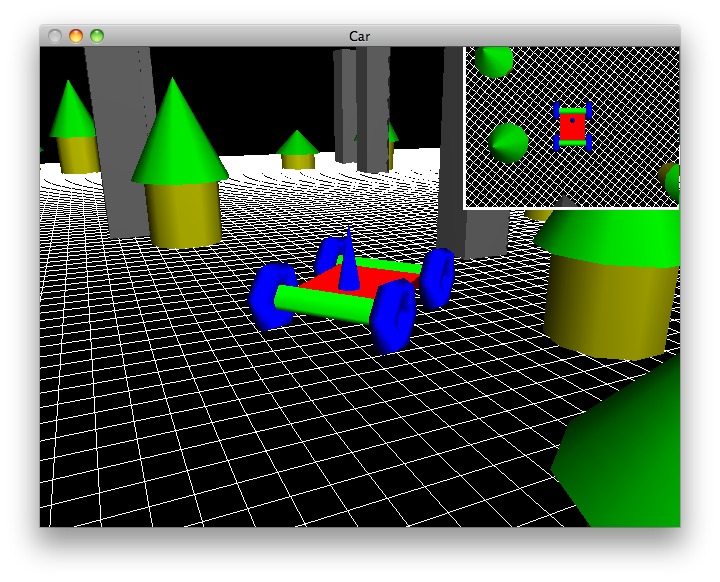 Today's aerial coverage brought to you by Goodyear!
You have now made up your mind and thank the man. He rejoins his group and you watch them travel out of sight. Once they pass, you continue along
the road hoping to meet others in your quest.
Part II - Website
Update the webpage that you submitted with A2 to include an entry for this assignment. As
usual, include a screenshot (or two) and a brief description of the program, intended to showcase what
your program does to people who are not familiar with the assignment.
Documentation
With this and all future assignments, you are expeced to appropriately document your code. This includes
writing comments in your source code - remember that your comments should explain what a piece of code
is supposed to do and why; don't just re-write what the code says in plain English. Comments serve the dual
purpose of explaining your code to someone unfamiliar with it and assisting in debugging. If you know
what a piece of code is supposed to be doing, you can figure out where it's going awry more easily.
Proper documentation also means including a README.txt file with your submission. In your
submission folder, always include a file called README.txt that lists:
- Your Name / email
- Assignment Number / Project Title
- A brief, high level description of what the program is / does
- A usage section, explaining how to run the program, which keys perform which actions, etc.
- Instructions on compiling your code
- Notes about bugs, implementation details, etc. if necessary
- How long did this assignment take you?
- How much did the lab help you for this assignment? 1-10 (1 - did not help at all, 10 - this was exactly the same as the lab)
- How fun was this assignment? 1-10 (1 - discontinue this assignment, 10 - I wish I had more time to make it even better)
Grading Rubric
Your submission will be graded according to the following rubric.
10% | Character must be constructed in a hierarchical fashion. Character MUST be broken down into function calls that handle smaller components. |
10% | Some portion of the character is animated while moving (arms & legs swinging, wheels spinning, turret rotating, etc.). It can also be animated when still, but some aspect of animation must be keyed to the movement. |
10% | User must be able to move the character forward and backwards along its heading with the keyboard. Character stays on the grid and cannot move beyond its boundaries. |
10% | User must be able to change the character's heading with the keyboard. |
5% | Character must rotate appropriately with heading change. |
5% | There must be some "scene" surrounding the character to provide a sense of motion when the character moves. This must include a grid and possibly a collection of decorative objects. |
5% | Character must use different colors for different parts. |
5% | Character must be comprised of 3D GLUT primitives / GLU quadrics. |
10% | The camera must follow an arcball camera model, have the character as its look-at point, and must move with the character. |
10% | The user must be able to correctly control the angles of the camera with the mouse by holding the left mouse button and dragging. |
10% | The user must be able to correctly zoom in and out by holding CTRL + left mouse button and dragging. |
5% | Submission includes source code, Makefile, and README.txt. Source code is well documented. Webpage named <afsid>.html submitted and updated with screenshot from latest assignment. |
5% | Submission compiles and executes in the lab machine environment. |
For students in CSCI441: To earn the extra credit achievement, the following criteria must be met. Late submissions are ineligible to earn the extra credit achievement.
For students in CSCI598B: This section will count as an additional 50 points, with the 50 points being broken down as follows.
20% | The viewport is drawn correctly for the secondary display. |
20% | The viewport for the original display is not adversely affected by the secondary display's viewport (i.e. viewport is reset properly, there are no related bugs). |
20% | The user must be able to render the scene from a secondary virtul camera. |
20% | The secondary view must be a camera attached to the vehicle, whose orientation is fixed to the vehicle. |
20% | The scene must be more complicated than a single ground plane; it must include multiple objects scattered around to give a sense of motion as the vehicle navigates. |
Experience Gained & Available Achievements
Assignments +100 XP
|
Web +100 XP
|
Mercator
|
Doom iddt
|
Submission
Please update your Makefile so it produces an executable with the name a3. When you are completed with the assignment, zip together your source code, Makefile, README.txt, and www/ folder. Name the zip file, HeroName_A3.zip. Upload this file to Blackboard under A3.
This assignment is due by Friday, September 16, 2016 by 11:59pm.
|