HOMEWORK 03 - ROCK, PAPER, SCISSORS
Concepts
In this homework assignment you have the opportunity to experiment with boolean expressions and conditional statements.
Before you Begin Coding
It's easy for the logic in this assignment to get out of control very quickly. We strongly recommend that you sit down with a pencil and paper to sketch the flow of the logic that you'll need. Use pseudocode, rough flowcharts, boxes, arrows, stickmen, or whatever means you need to create a clear structure for the logic of your Rock, Paper, Scissors game.
After you have pseudocode for your solution, then create yourself an empty project. You should know how to do this by now.
RPS
Most of you have likely played the classic game Rock, Paper, Scissors. Believe it or not, a hardcore world of Rock, Paper, Scissors has existed, even in Denver. It's a bit frightening.... So, before you start thinking how Rock, Papers, Scissors (or RPS for those in the know) is a kid's game, think again. This is serious stuff; go ahead and TRY to beat the computer on this NY Times link. (But, if anyone asks, your C++ homework this week is to implement a simple interactive rule-based system that addresses a theoretical Decision Problem.)
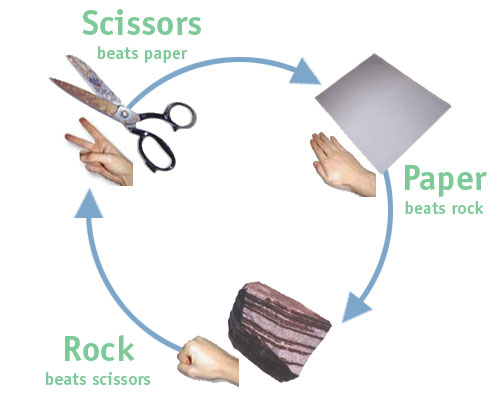
Instructions: Step I
Your goal is to implement a two-player version of Rock, Paper, Scissors. Players will enter either R or r for rock, P or p for paper, and S or s for scissors. Your program must then simply announce the winner. Note that your program only needs to play one round of the game. We will add repetition via loops next week. Here is an example interaction:
Player one: R
Player two: s
Rock beats scissors. Player one wins!
Here is another example:
Player one: p
Player two: S
Scissors beats paper. Player two wins!
Your program must only prompt for two inputs. It should also print a line following this pattern:
Where X and Y are one of "rock" or "scissors" or "paper" and Z is either "one" or "two".
Yes, we (and the RPS Society) understand that this interactive game is a little silly since Player two can always see what Player one chooses. Let's fix this issue next (AFTER you have this silly initial game working).
Instructions: Step II
Now that you have your RPS game working correctly, modify your code to let the computer generate a random number for Player 2. Specifically, generate a random number and then use (randNumGenerated % 3) to generate a 0 (rock), 1 (paper), or 2 (scissors) for Player 2. (Then assign 'r', 'p', or 's' to Player 2's choice accordingly.)
Example outputs follows:
Player one: R
Computer: s
Rock beats Scissors. Player one wins!
Here is another example:
Player one: R
Computer: p
Paper beats rock. Computer wins!
The solution you submit should provide output like the last two example outputs (the two framed in black).
Submission
You need to submit your solution of this homework (HW03) with two lab assignments: Lab03A and Lab03B. Detailed generic instructions for submitting homework assignments are available. For homework due this Wednesday, follow these specific steps:
- create a directory called week03.
- within week03, create three subdirectories: Lab03A, Lab03B, and HW03.
- within your new week03/Lab03A directory, copy in your main.cpp file from your Lab03A solution.
- within your new week03/Lab03B directory, copy in your main.cpp file from your Lab03B solution.
- within your new week03/HW03 directory, copy in your main.cpp file from your HW03 solution.
- compress the week03 directory (see Step 3 here for details).
- submit the week03.zip file to Blackboard (see Steps 5-10 here for details).
- after you submit, download the file and double check it contains all that you think it contains!